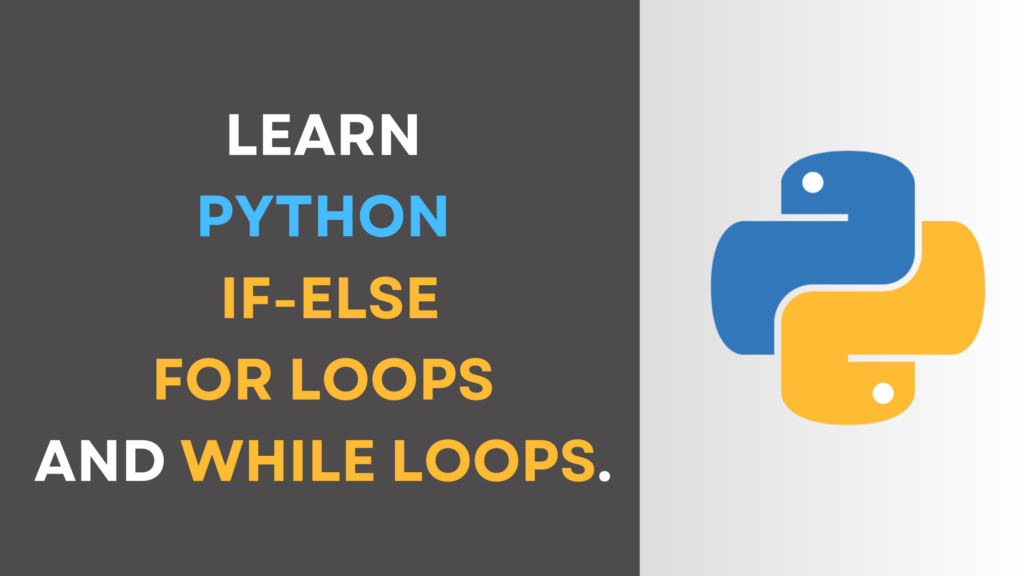
Welcome to the world of Python programming! One of the foundational concepts you’ll encounter on your journey is control flow. Don’t worry if you’re new to programming; we’re here to guide you through the essential control flow statements in Python: if-else, for loops, and while loops.
In this beginner-friendly article, we’ll break down these concepts into bite-sized pieces with easy-to-understand examples, empowering you to wield Python’s control flow like a pro.
Here we discussed all about
Learn Python programming if-else, for loops, and while loops.
Understanding If-Elif-Else Statements:
If-else statements are used to make decisions in your code based on certain conditions.
1. “if” statement:
In programming, an “if” statement allows us to execute a certain block of code only if a specific condition is true. Here’s a closer look at how it works in Python:
i. Check if a number is positive:
Explanation: In the example, the code checks if the variable num is greater than 0. If it is, it prints “Number is positive.”.
num = 7
if num > 0:
print("Number is positive.")
# output: Number is positive.
ii. Check if a number is even:
Explanation: In the example, the code uses the modulo operator (%) to check if the remainder of num divided by 2 is equal to 0. If it is, the number is even, so it prints “Number is even.”
num = 8
if num % 2 == 0:
print("Number is Even")
# output: Number is Even
iii. Check if a number is between a range:
The code checks if num
falls between 10 and 20 exclusive. If it does, it prints “Number is between 10 and 20”.
num = 15
if 10 < num < 20:
print("Number is between 10 and 20")
# output: Number is between 10 and 20
iv. Check if a string is empty:
In the example, the not
keyword checks if str
is empty. If it is, it prints “String is empty”.
str = ""
if not str:
print("String is empty")
# output: String is empty
v. Check if a list is not empty:
The code checks if my_list
contains any elements. If it does, it prints “List is not empty”.
my_list = [1,2,3]
if my_list:
print("List is not empty.")
# output: List is not empty
vi. Check if a character is a vowel:
It checks if the character stored in char
is a vowel (present in the string ‘dear’). If it is, it prints “Character is a vowel”.
char = "a"
if char in "dear":
print("Charater is a vowel")
# output: Charater is a vowel
vii. Check if a number is within a list:
Explanation: The code checks if num
is present in the list my_list
. If it is, it prints “Number is in the list”.
num = 8
my_list = [1,2,4,6,7,8,9]
if num in my_list:
print("Number is in the list")
# output: Number is in the list
viii. Check if a user’s age meets a certain requirement:
Explanation: The code checks if the variable age
is greater than or equal to 18. If it is, it prints “User is an adult”.
age = 25
if age >= 18:
print("User is an Adult.")
# output: User is an Adult.
ix. Check if two strings are equal:
Explanation: The code checks if str1
is equal to str2
. If they are equal, it prints “Strings are equal”.
str1 = "hello"
str2 = "hello"
if str1 == str2:
print("Strings are equal")
# output: Strings are equal
x. Check if a number is negative or zero:
Explanation: The code checks if num
is less than or equal to 0. If it is, it prints “Number is negative or zero”.
num = -5
if num <= 0:
print("Number is negative or zero")
# output: Number is negative or zero
2. If-else statement:
if-else statement” in Python is used to execute different blocks of code based on whether a condition is true or false. It consists of the if
keyword followed by a condition, and optionally followed by an else
keyword and another block of code.
Here are 10 basic examples to understand the Python if-else statement.
i. Check if a number is even or odd:
If the remainder of num
divided by 2 equals 0, it’s even; otherwise, it’s odd.
num = 7
if num % 2 == 0:
print("Number is even")
else:
print("Number is odd")
# output: Number is odd
ii. Determining whether a person is eligible to vote or not:
If the age
is greater than or equal to 18, the person is eligible to vote; otherwise, they are not.
age = 20
if age >= 18:
print("You are eligible to vote")
else:
print("You are not eligible to vote")
# output: You are eligible to vote
iii. Comparing two numbers and printing the larger one:
If num1
is greater than num2
, it prints num1
as the larger number; otherwise, it prints num2
.
num1 = 20
num2 = 30
if num1 > num2:
print("The larger number is: ", num1)
else:
print("The larger number is: ", num2)
# output: The larger number is: 30
iv. Determining if a year is a leap year or not :
If the year
is divisible by 4 but not by 100, or if it’s divisible by 400, then it’s a leap year; otherwise, it’s not.
year = 2024
if (year % 4 == 0 and year % 100 != 0) or (year % 400 == 0):
print(year, "is a leap year")
else:
print(year, "is not a leap year")
# output: 2024 is a leap year
v. Checking if a number is within a specified range or not:
Explanation: If num
falls within the range of 20 to 30 (inclusive), it prints that it’s within the range; otherwise, it prints it’s outside the range.
num = 25
if 20 <= num <= 30:
print("Number is within the range of 20 to 30")
else:
print("Number is outside the range.")
# output: Number is within the range of 20 to 30
vi. Determining the pass and fail based on the percentage:
If percentage
is greater than or equal to 30, then it print ‘You are pass’ otherwise, it prints “You are fail”.
percentage = 40
if percentage >= 30:
print("You are pass")
else:
print("You are fail")
# output: You are pass
vii. Checking if a number is positive or negative:
It checks whether num
is positive or negative and prints the appropriate message accordingly.
num = -5
if num > 0:
print("Number is positive")
else:
print("Number is Negative")
# output: Number is Negative
viii. How to figure out if a year is a century year or not:
If the year
is divisible by 100, then it’s a century year; otherwise, it’s not.
year = 2000
if year % 100 == 0:
print(year, "is a century year")
else:
print(year, "is not a century year")
# output: 2000 is a century year
ix. Checking if a string is empty or not:
If str
has any characters (i.e., it’s not empty), it prints that the string is not empty; otherwise, it prints that the string is empty.
str = "Hello"
if str:
print("String is not empty")
else:
print("String is empty")
# output: String is not empty
x. Checking if a year is a future year or not:
It compares year_to_check
with the current year. If year_to_check
is greater than the current year, it prints that it’s a future year; otherwise, it prints that it’s not a future year.
import datetime
current_year = datetime.datetime.now().year
year_to_check = 2050
if year_to_check > current_year:
print(year_to_check, "is a future year")
else:
print(year_to_check, "is not a future year")
# output: 2050 is a future year
3. If-elif-else statement:
The “if-elif-else” statement in Python is an extension of the “if-else” statement. It allows you to handle multiple conditions sequentially.
The syntax is as follows:
if condition1:
# Code block to execute if condition1 is True
elif condition2:
# Code block to execute if condition1 is False and condition2 is True
elif condition3:
# Code block to execute if both condition1 and condition2 are False and condition3 is True
…
else:
# Code block to execute if all conditions are False
Here are 10 basic examples to understand the Python if-elif-else statement.
i. Categorizing numbers as positive, negative, or zero:
Categorize a number as positive, negative, or zero based on its value.
num = 0.9
if (num > 0):
print("Number is positive")
elif (num < 0):
print("Number is negative")
else:
print("Number is zero")
# output: Number is positive
ii. Finding the greatest number between three numbers:
It compares three numbers and prints the largest among them.
num1 = 10
num2 = 30
num3 = 25
if num1 > num2 and num1 > num3:
print(num1, "is the largest number.")
elif num2 > num1 and num2 > num3:
print(num2, "is the largest number.")
else:
print(num3, "is the largest number.")
# output: 30 is the largest number.
iii. Calculating the grade using the percentage:
It assigns a grade based on the percentage obtained.
percentage = 76
if percentage >= 90:
print("Grade: A")
elif percentage >= 80:
print("Grade: B ")
elif percentage >= 70:
print("Grade: C")
elif percentage >= 60:
print("Grade: D")
else:
print("Grade: F")
# output: Grade: C
iv. Identifying whether a triangle is scalene, isosceles, or equilateral:
It determines the type of triangle based on the lengths of its sides.
a = 5
b = 5
c = 5
if a == b == c:
print("Equilateral triangle")
elif a == b or b == a or c == a:
print("Isosceles triangle")
else:
print("Scalene triangle")
# output: Equilateral triangle
v. Making Decisions Based on Age:
Here, Python decides what activity to do based on your age.
age = 7
if age < 6:
print("Play with toys!")
elif age <= 12:
print("Play video games!")
else:
print("Read books!")
# output: Play video games!
vi. Using multiple conditions to print positive and even, and positive, odd, and negative numbers:
It combines conditions using logical operators (and
).
x = 10
if x > 0 and x % 2 ==0:
print(x,"is positive and even")
elif x > 0:
print(x,"is positive but odd")
else:
print(x,"is negative")
# output: 10 is positive and even
vii. Ticket Price Calculator:
This program calculates the ticket price based on age.
# Ticket price calculator
age = int(input("Enter your age: "))
if age < 6 or age >= 60:
print("Ticket price: Free")
elif 6 <= age <= 17:
print("Ticket price: Rs 700")
else:
print("Ticket price: Rs 1500")
viii. Password Strength Checker:
This program checks the strength of a password based on its length.
password = input("Enter your password: ")
if len(password) >= 16:
print("Strong Password")
elif len(password) >= 8:
print("Medium Password")
elif len(password) >= 5:
print("Weak Password")
else:
print("Sorry! Easy password not allowed")
ix. Simple Calculatorr:
This program performs arithmetic operations based on user input.
num1 = float(input("Enter the first number: "))
num2 = float(input("Enter the second number: "))
operation = input("Enter the operation (+, -, *, /): ")
if operation == '+':
print("Result:", num1 + num2)
elif operation == '-':
print("Result:", num1 - num2)
elif operation == '*':
print("Result:", num1 * num2)
elif operation == '/':
if num2 != 0:
print("Result:", num1 / num2)
else:
print("Cannot divide by zero!")
else:
print("Invalid operation")
x. Divisibility Checker:
number = int(input("Enter a number: "))
if number % 2 == 0:
print("Number is divisible by 2.")
elif number % 3 == 0:
print("Number is divisible by 3.")
elif number % 5 == 0:
print("Number is divisible by 5.")
else:
print("Number is not divisible by 2, 3, or 5.")
4. For Loops:
For every element in a sequence, a Python for loop executes a block of code, It is a control flow statement that is frequently used when you want to repeat a task on every item in a collection, such as a list, tuple, string, or range
Here are 10 basic examples to understand the Python for loop.
i. Print numbers from 1 to 7 using the for loop:
This program uses the range()
function to generate numbers from 1 to 7 (inclusive). The for loop iterates over each number in this range and prints it.
for i in range(1,8):
print(i)
# output:
1
2
3
4
5
6
7
ii. Print even numbers from 1 to 12 using the for loop:
This program uses the range()
function with a step of 2 to generate even numbers from 2 to 12. The for loop then iterates over each even number and prints it.
for i in range(2,13,2):
print(i)
# output:
2
4
6
8
10
12
iii. Calculate the sum of numbers from 1 to 10 using the for loop:
This program calculates the sum of numbers from 1 to 10 using a for loop. It initializes a variable total
to 0 and adds each number from 1 to 10 to total
inside the loop.
total = 0
for i in range(1,10):
total += i
print("Sum of numbers:",total)
# output: Sum of numbers: 45
iv. Print Characters of a String using the for loop:
This program iterates over each character in the string “Python” and prints each character on a separate line.
word = "Python"
for char in word:
print(char)
# output:
P
y
t
h
o
n
v. Print List Elements using the for loop:
This program iterates over each element in the list fruits
and prints each element (fruit) on a separate line.
fruits = ["Banana","Apple","PineApple","Cherry","Mango"]
for fruit in fruits:
print(fruit)
# output:
Banana
Apple
PineApple
Cherry
Mango
vi. Find the Maximum Number in a List using the for loop:
This below program finds the maximum number in the list numbers
using a for loop and an if
statement to compare each number with the current maximum.
numbers = [1,23,12,15,2]
max_number = numbers[0]
for num in numbers:
if num > max_number:
max_number = num
print("Maximum number:", max_number)
output: Maximum number: 23
vii. Print Table of 2 using the for loop:
This program prints the table of 2 by iterating from 1 to 10 and multiplying each number by 2 inside the loop.
for i in range(1, 11):
print("2 *",i, "=", 2*i)
output:
2 * 1 = 2
2 * 2 = 4
2 * 3 = 6
2 * 4 = 8
2 * 5 = 10
2 * 6 = 12
2 * 7 = 14
2 * 8 = 16
2 * 9 = 18
2 * 10 = 20
viii. Calculate Factorial of a Number using the for loop:
This program calculates the factorial of a number (in this case, 5) using a for loop and the multiplication operator.
number = 5
factorial = 1
for i in range(1, number + 1):
factorial *= i
print("Factorial of", number, "is",factorial)
# output: Factorial of 5 is 120
ix. Calculate Simple Interest using the for loop:
This program calculates simple interest using the formula Simple Interest = Principal * Rate * Time
. It demonstrates how a for loop is not always necessary for every program, as in this case, where we perform a simple calculation without iteration.
principal = 2000
rate = 0.1
time = 2 # years
simple_interest = principal * rate * time
print("Simple Interest:", simple_interest)
# output: Simple Interest: 400.0
x. Program to reverse a string using the for loop:
This program reverses the string “Hello World!” using a for loop. It iterates over each character in the string and appends it to the beginning of reversed_text
, effectively reversing the string.
text = "Hello World!"
reversed_text = ""
for char in text:
reversed_text = char + reversed_text
print("Reversed:", reversed_text)
# output: Reversed: !dlroW olleH
x. This program calculates the average of numbers in a list using for loop:
numbers = [10,20,30,40,50,60]
total = 0
for num in numbers:
total += num
average = total / len(numbers)
print("Average:", average)
output: Average: 35.0
4. While Loops:
A while loop in Python is another type of loop that allows you to execute a block of code repeatedly as long as a specified condition is true. It’s useful when you want to repeat a task until a certain condition is met, and you may not know in advance how many times the loop will need to iterate.
Here are 10 basic examples to understand the Python while loop.
i. Counting Numbers using while loop:
This program uses a while loop to count from 1 to 5. It initializes a variable count
to 1 and continues printing the value of count
while it is less than or equal to 5. The count
variable is incremented by 1 in each iteration.
count = 1
while count <= 5:
print(count)
count +=1
# output:
1
2
3
4
5
ii. Print Even Numbers using while loop:
This program prints even numbers from 2 to 10 using a while loop. It initializes a variable num
to 2 and continues printing num
while it is less than or equal to 10. The num
variable is incremented by 2 in each iteration to ensure only even numbers are printed.
num = 2
while num <= 10:
print(num)
num += 2
# output:
2
4
6
8
10
iii. Calculate Factorial using while loop:
This program calculates the factorial of a number (in this case, 5) using a while loop. It initializes variables factorial
and i
to 1 and iterates from 1 to the given number, multiplying each number by the factorial
variable.
number = 5
factorial = 1
i = 1
while i <= number:
factorial *= i
i += 1
print("Factorial of", number, "is", factorial)
iv. Print Table of 3 using while loop:
This program prints the table of 3 using a while loop. It initializes a variable num
to 1 and continues printing the result of 3 * num
while num
is less than or equal to 10. The num
variable is incremented by 1 in each iteration..
num = 1
while num <= 10:
print("3 * ", num, "=", 3* num)
num += 1
# output:
3 * 1 = 3
3 * 2 = 6
3 * 3 = 9
3 * 4 = 12
3 * 5 = 15
3 * 6 = 18
3 * 7 = 21
3 * 8 = 24
3 * 9 = 27
3 * 10 = 30
v. Password Checker using while loop:
This program checks the user’s password using a while loop. It continues prompting the user to enter the correct password until they get it right.
password = "password"
attempt = input("Enter the password: ")
while attempt != password:
print("Incorrect password. Try again.")
attempt = input("Enter the password: ")
print("Welcome, Access granted!")
vi. Countdown Timer using while loop:
This program implements a countdown timer using a while loop. It initializes a variable seconds
to 10 and prints the remaining seconds while seconds
is greater than 0, decrementing seconds
by 1 in each iteration.
seconds = 10
while seconds > 0:
print(seconds)
seconds -= 1
print("Time's up!")
vii. Print Alphabetss using while loop:
This program prints the uppercase alphabets from ‘A’ to ‘Z’ using a while loop. It initializes a variable char
to ‘A’ and continues printing char
while it is less than or equal to ‘Z’, incrementing char
by 1 in each iteration.
char = 'A'
while char <= "Z":
print(char, end=" ")
char = chr(ord(char) + 1)
# output: A B C D E F G H I J K L M N O P Q R S T U V W X Y Z
viii. Print Numbers in reverse using the while loop:
This program prints numbers from 10 to 1 in reverse order using a while loop. It initializes a variable num
to 10 and decrements it by 1 in each iteration.
num = 10
while num >= 1:
print(num)
num -= 1.
# output:
10
9
8
7
6
5
4
3
2
1
ix. Calculate Sum of Digits of 1234 using the while loop:
This program calculates the sum of digits of the number 1234 using a while loop.
num = 1234
total = 0
while num > 0:
total += num % 5
num //= 10
print("Sum of digits of 1234:", total)
x. Print Odd Numbers using the while loop:
This program prints odd numbers from 1 to 10 using a while loop.
num = 1
while num <= 10:
print(num)
num += 2
# output:
1
3
5
7
9